The Flutter
Micro-framework
For Modern Apps
A solid foundation for building Flutter apps.
Get Started!
Develop your next Flutter app with Nylo
Nylo is a powerful framework for developing mobile apps in Flutter. Out the box it comes with a router, secure storage, networking and more.
Download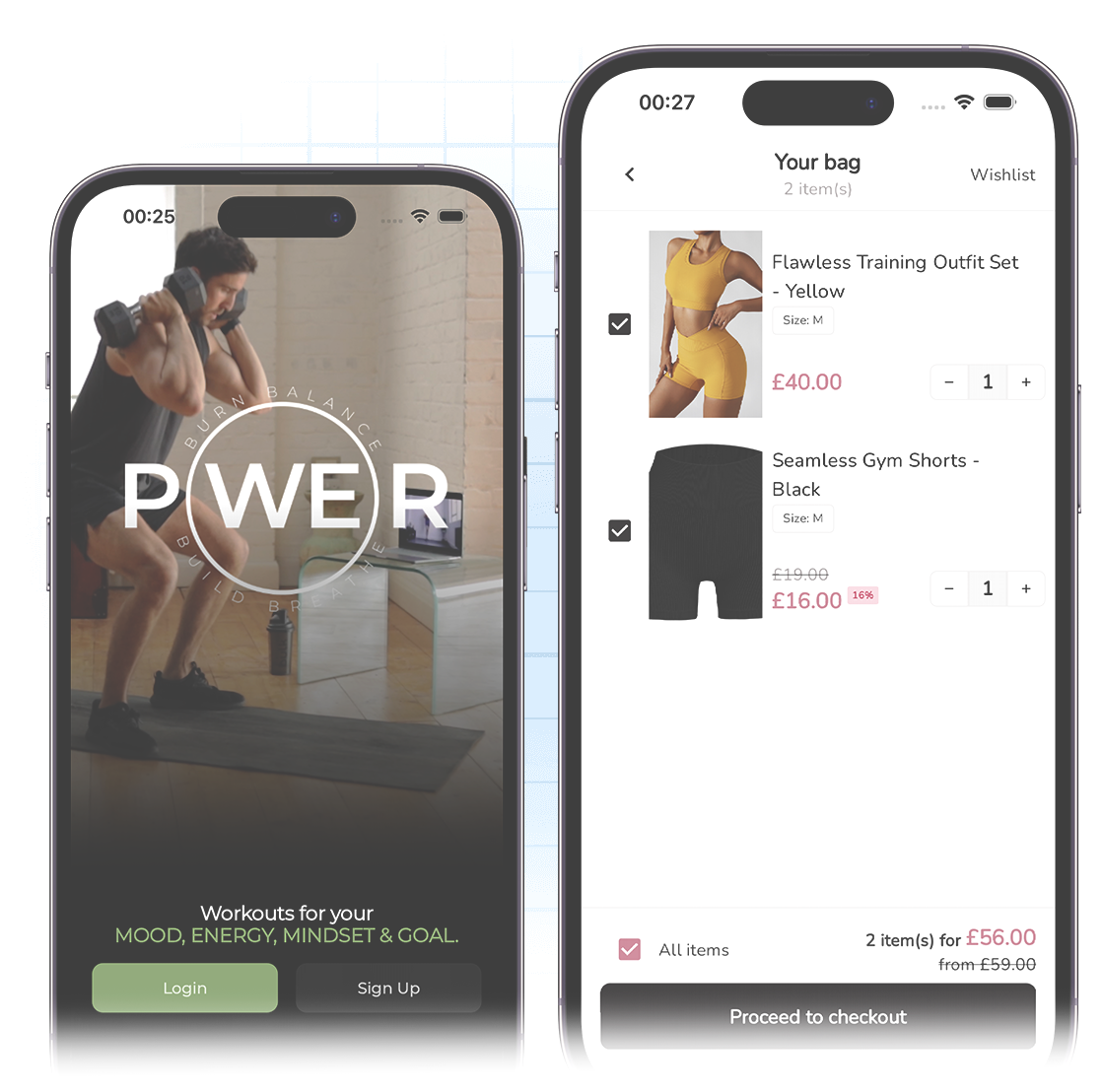
Powerful tools for creating
Everything you need to build your next Flutter app
- Routing
- Authentication
- Forms
- Navigation Hub
- State Management
- Events
- Commands
- Scheduler
appRouter() => nyRoutes((router) {
router.add(HomePage.path).initialRoute();
router.add(DiscoverPage.path);
router.add(LoginPage.path,
transitionType: TransitionType.bottomToTop());
router.add(ProfilePage.path,
routeGuard: [
AuthGuard()
]
);
});
Build complex routes, interfaces and UI pages for your Flutter application.
Learn more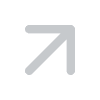
Authenticate a user
String userToken = "eyJhbG123...";
await Auth.authenticate(data: {"token": userToken});
Now, when your user opens the app they will be authenticated.
final userData = Auth.data();
// {"token": "eyJhbG123..."}
bool isAuthenticated = await Auth.isAuthenticated();
// true
If you've set an authenticatedRoute in your router, then it will present this page when the user opens the app again.
appRouter() => nyRoutes((router) {
...
router.add(LandingPage.path).initialRoute();
router.add(DashboardPage.path).authenticatedRoute();
// overrides the initial route when a user is authenticated
Logout the user
await Auth.logout();
Authenticate users in your Flutter application.
Learn more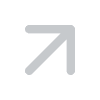
Create a Form
metro make:form RegisterForm
Modify your form
class RegisterForm extends NyFormData {
RegisterForm({String? name}) : super(name ?? "login");
// Add your fields here
@override
fields() => [
Field.capitalizeWords("name",
label: "Name",
validator: FormValidator.notEmpty(),
),
Field.email("email_address",
label: "Email",
validator: FormValidator.email()
),
Field.password("password",
label: "Password",
validator: FormValidator.password(),
),
];
@override
Widget? get submitButton => Button.primary(text: "Submit", submitForm: (this, (data) {
print(["formData", data]);
}));
}
Use your form in a widget
AdvertForm form = AdvertForm();
@override
Widget build(BuildContext context) {
return Scaffold(
body: NyForm(form: form),
);
}
// or like this
AdvertForm form = AdvertForm();
@override
Widget build(BuildContext context) {
return Scaffold(
body: NyForm(form: form, footer: Button.primary(text: "Submit", submitForm: (form, data) {
print(["formData", data]);
})),
);
}
Manage, validate and submit data all in one place with Nylo Forms.
Learn more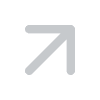
Create a state managed widget
metro make:stateful_widget CartIcon
class _CartIconState extends NyState<CartIcon> {
String? _cartValue;
@override
void stateUpdated(data) {
_cartValue = data;
// data is passed from the updateState method
setState(() {});
}
@override
Widget build(BuildContext context) {
return Badge(
child: Icon(Icons.shopping_cart),
label: Text(_cartValue ?? "1"),
);
}
}
Now, you can update this widget from anywhere in your application
Use the updateState method like in the below code snippet
Button.primary(text: "Add to cart",
onPressed: () {
updateState(CartIcon.state, data: "2");
}
)
You can pass any object to the widget
Powerful state management for widgets in your Flutter application.
Learn more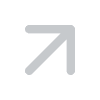
Create a Navigation Hub widget
metro make:navigation_hub base
...
class _BaseNavigationHubState extends NavigationHub<BaseNavigationHub> {
/// Layouts:
/// - [NavigationHubLayout.bottomNav] Bottom navigation
/// - [NavigationHubLayout.topNav] Top navigation
/// - [NavigationHubLayout.journey] Journey navigation
NavigationHubLayout? layout = NavigationHubLayout.bottomNav(
// backgroundColor: Colors.white,
);
/// Should the state be maintained
@override
bool get maintainState => true;
/// Navigation pages
_BaseNavigationHubState() : super(() async {
return {
0: NavigationTab(
title: "Home",
page: HomeTab(),
icon: Icon(Icons.home),
activeIcon: Icon(Icons.home),
),
1: NavigationTab(
title: "Settings",
page: SettingsTab(),
icon: Icon(Icons.settings),
activeIcon: Icon(Icons.settings),
),
};
});
}
NavigationHubLayout.topNav
class _BaseNavigationHubState extends NavigationHub<BaseNavigationHub> {
NavigationHubLayout? layout = NavigationHubLayout.topNav(
...
);
}
Create your event
metro make:event Logout
class LogoutEvent implements NyEvent {
@override
final listeners = {
DefaultListener: DefaultListener(),
};
}
class DefaultListener extends NyListener {
@override
handle(dynamic event) async {
// logout user
await Auth.logout();
// redirect to home page
routeTo(HomePage.path,
navigationType: NavigationType.pushAndForgetAll
);
}
}
Dispatch the event
MaterialButton(child: Text("Logout"),
onPressed: () {
event<LogoutEvent>();
},
)
Dispatch events and listen for them in your application.
Learn more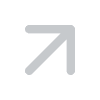
Create a Command
metro make:command CurrentTime
Modify your command
class _CurrentTimeCommand extends NyCustomCommand {
_CurrentTimeCommand(super.arguments);
@override
CommandBuilder builder(CommandBuilder command) {
command.addOption("format", defaultValue: "HH:mm:ss");
return command;
}
@override
Future<void> handle(CommandResult result) async {
final format = result.getString("format");
final DateFormat dateFormat = DateFormat(format);
// Format the current time
final formattedTime = dateFormat.format(DateTime.now());
info("The current time is " + formattedTime);
}
}
Run your command
metro app:current_time
Create custom commands within your project
Learn more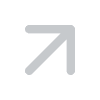
Schedule a task to run once
Nylo.scheduleOnce("onboarding_info", () {
print("Perform code here to run once");
});
Schedule a task to run once after a specific date
Nylo.scheduleOnceAfterDate("app_review_rating", () {
print("Perform code to run once after DateTime(2025, 04, 10)");
}, date: DateTime(2025, 04, 10));
Schedule a task to run once daily
Nylo.scheduleOnceDaily("free_daily_coins", () {
print("Perform code to run once daily");
});
Schedule tasks to run once or daily in your Flutter application.
Learn more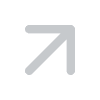
We have built a cli tool called Metro, you can create almost anything on the fly.
Learn more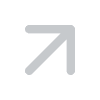
metro make:page HomePage
# Creates a new page called HomePage
metro make:api_service User
# Creates a new API Service called UserApiService
metro make:model User
# Creates a new model called User
metro make:stateful_widget FavouriteWidget
# Creates a new stateful widget called FavouriteWidget
class ApiService extends NyApiService {
@override
String get baseUrl => "https://api.example.com/v1";
Future<List<Post>> posts() async {
return await network(
request: (request) => request.get("/posts"),
);
}
}
...
class _HomePageState extends NyState<HomePage> {
@override
get init => () async {
final posts = await api<ApiService>((request) => request.posts());
};
Nylo's Community
Together, we can build better Flutter apps.
“I’m new to Dart and new to your framework (which I love)”
Peter
Senior Director of Heroku Global
“I wanted to thank you guys for the great job you are doing.”
@youssefKadaouiAbbassi
“Just to say that I am in love with @nylo_dev's website!! I mean, look at this: https://nylo.dev Definitely gonna explore it!”
@esfoliante_txt
“Really love the concept of this framework”
@Chrisvidal
“Just discovered http://nylo.dev and looking forward using in production.”
@medilox
“Nice Framework”
@ChadereNyore
"Nylo" is the best framework for flutter, which make it developing easy such as, file-structure, routing, state, color theme and so on.
@higakijin
This is incredible. Very well done!
FireflyDaniel
Big support and love from morocco <3
@imlian
Very nice Framework! Thank you so much!
@ChaoChao2509
I just discover this framework and i'm verry impressed. Thank you
@lepresk
Great work on Nylo
@dylandamsma